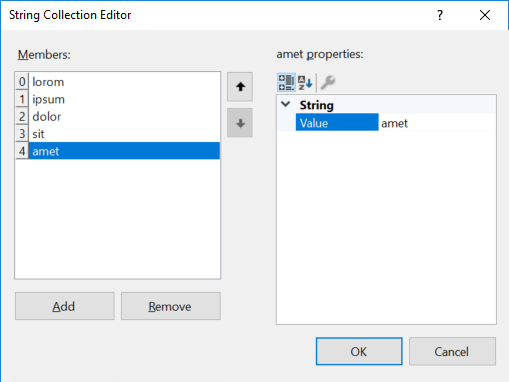
When you define a property of List<string>
type for a control, if you try to edit the property in PropertyGrid
, a collection editor form will open and if you click on add button on the editor form, you will face with an error:
Constructor on type ‘System.String’ not found.
How can we edit the list in a collection editor form without facing such error message? Is there any UITypeEditor
suitable to edit List<string>
without problem? In this post, I’ll show how you can edit a List<string>
in property grid without any problem.
Let’s say we have a control containing the following property:
private List<string> myList = new List<string>();
public List<string> MyList {
get {
return myList;
}
set {
myList = value;
}
}
If it was string[]
we were safe and without doing anything special, the property grid would show a standard dialog containing a multi-line text box to edit string array and each line will be an element in the array. But the case for List<string>
is different.
To edit List<string>
in property grid, you can use either of the following options:
StringCollectionEditor
which shows a dialog containing a multi-line text box to edit elements- Create a custom
CollectionEditor
to edit items in a collection editor dialog
Option 1 – StringCollectionEditor
private List<string> myList = new List<string>();
[Editor("System.Windows.Forms.Design.StringCollectionEditor, " +
"System.Design, Version=2.0.0.0, Culture=neutral, PublicKeyToken=b03f5f7f11d50a3a",
typeof(UITypeEditor))]
[DesignerSerializationVisibility(DesignerSerializationVisibility.Content)]
public List<string> MyList {
get {
return myList;
}
set {
myList = value;
}
}
Option 2 – Custom CollectionEditor
First create the custom editor:
//You need to add reference to System.Design
public class MyStringCollectionEditor : CollectionEditor {
public MyStringCollectionEditor() : base(type: typeof(List<String>)) { }
protected override object CreateInstance(Type itemType) {
return string.Empty;
}
}
Then decorate the property with the editor attribute:
private List<string> myList = new List<string>();
[Editor(typeof(MyStringCollectionEditor), typeof(UITypeEditor))]
[DesignerSerializationVisibility(DesignerSerializationVisibility.Content)]
public List<string> MyList {
get {
return myList;
}
set {
myList = value;
}
}
Thank you so much, Simple n Clean
Nice solution. Thanks. How simple. 🙂
How can i save multiple variables of diffrent type string,int,bool,list etc. to be used as application configuration to a table and retrive each time application starts from table and save back if user want to change them. earlier i was using app.config but with every build for diffrent users it becomes useless and lost. it its saved in a table it will remain consistent.